I think this subject probably gets asked a lot by most new Flex programmers. Unfortunately, most of the companies I have worked for did little to enforce or even develop coding standards. Larger companies typically make it a priority to develop standards simply due to the number of incoming and outgoing contractors hired for specific projects. Lack of standards can cause code nightmares later on and virtually no project maintainability.
A good starting point for standards can be from Adobe themselves, who have come up with a nice document detailing basic Flex coding conventions and best practices. See for yourself!
http://opensource.adobe.com/wiki/display/flexsdk/Coding+Conventions
Adobe Flex has been out for about five years now and although it is still quite young, a handful of printed magazines have arisen to extol the virtues of this powerful platform.
Someone asked if I could create a talk or thought bubble component similar to talk bubbles in cartoons. Of course, not having done this sort of thing before I started going over the possible ways of doing such a thing in my head.
- Create a “skin” in Photoshop or Illustrator, embed that skin in a custom component or in a stylesheet and go from there
- Customize the ToolTip Manager somehow to get it to do what I want
- Create a custom component in Flex that used the ActionScript graphics API to draw the talk bubble
There might have been other ways but these were the first that came to mind. I realized I needed the talk bubble to expand or shrink depending on its textual (or non-textual) content. And I needed it to be easy for other developers to drop right into their applications without too much hassle. So I settled on #3, and after a mere two hours of work came up with this idea:
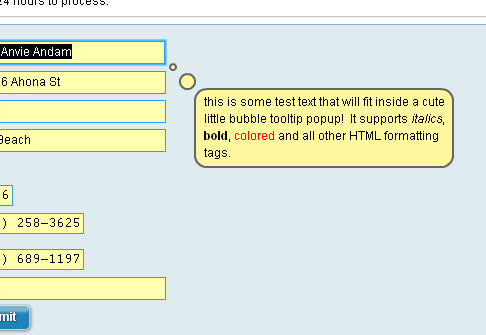
Talk Bubble ToolTip (down direction)
From the code snippet below you can see I simply created a new component in ActionScript which extended the Text component within the Flex SDK. I ensured that the visible and includeInLayout properties were set to false so that the bubble tooltip would only appear when the view set those values to true, say on a focus or mouse-over event.
Then, using the ActionScript graphics API, I drew three (3) rounded rectangles with the third’s dimensions calculated based on the textField’s content. The TextField itself is an inherited property of Text.as. I had to move the x & y coordinates of the textField so that it fit right over the third rectangle. In all, this gives us the appearance of a talk bubble! (sample example of usage is in the comments)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
| /**
* BubbleToolTip is a simple all-inclusive textual component that, when visible, will
* display a "thought bubble" tool-tip container wherever the tag is placed within a layout
* container (VBox, HBox, FormItem, etc).
*
* This extends mx.controls.Text so all properties and methods available via Text.as are
* available here.
*
* Sample Usage:
*
* <mx:FormItem id="emailForm"
* <mx:TextInput id="inpEmailAddress"
* focusOut="bubbleToolTip.visible = false"
* focusIn="bubbleToolTip.visible = true"/>
*
* <components:BubbleToolTip id="bubbleToolTip"
* x="{inpEmailAddress.x + inpEmailAddress.width}"
* y="{inpEmailAddress.y}"
* width="250" direction="up"/>
* </mx:FormItem>
*
*
* Sample Style:
*
* BubbleToolTip
* {
* background-alpha : 0.85;
* background-color : #FFF79F;
* border-color : #666666;
* color : #000000;
* font-family : Arial;
* font-size : 12px;
* }
*
*
* @author Erich Cervantez
* @date 01/13/2009
*/
package
{
import mx.controls.Text;
public class BubbleToolTip extends Text
{
/**
* Specifies the padding between text and bubble border
*/
private const PADDING : Number = 8;
/**
* @private
*/
private var m_direction : String;
/**
* Specifies the direction (up,down) of the bubble
*/
[Inspectable(category="General", enumeration="up,down", defaultValue="down")]
public function get direction() : String
{
return m_direction;
}
public function set direction( value : String ) : void
{
m_direction = value;
}
/**
* Constructor
*/
public function BubbleToolTip()
{
super();
visible = false;
includeInLayout = false;
}
/**
* Draw the customized text container whenever updateDisplayList is triggered
*/
override protected function updateDisplayList( unscaledWidth:Number, unscaledHeight:Number ) : void
{
super.updateDisplayList( unscaledWidth, unscaledHeight );
textField.x = textField.x + ( m_direction == "down" ) ? 32 : 35;
textField.y = textField.y + ( m_direction == "down" ) ? 28 : -( textField.height + PADDING/2 );
var calHeight : Number = textField.height + PADDING;
var calWidth : Number = textField.width + PADDING;
x = x + 5;
this.graphics.clear();
this.graphics.beginFill( getStyle('backgroundColor'), 1 );
this.graphics.lineStyle(2, getStyle('borderColor'), 1);
this.graphics.drawRoundRect(0, 0, 6, 6, 24, 24);
this.graphics.endFill();
this.graphics.beginFill( getStyle('backgroundColor'), 1 );
this.graphics.lineStyle(2, getStyle('borderColor'), 1);
this.graphics.drawRoundRect(10, m_direction == "down" ? 10:-10, 15, 15, 24, 24);
this.graphics.endFill();
this.graphics.beginFill( getStyle('backgroundColor'), 1 );
this.graphics.lineStyle(2, getStyle('borderColor'), 1);
this.graphics.drawRoundRect(m_direction == "down" ? 25:30, m_direction == "down" ? 25:-calHeight,
calWidth, calHeight, 24, 24);
this.graphics.endFill();
}
}
} |
Again, this was based on just a couple of hours of work. If anyone has some suggestions or additions to this bubble tooltip component, please let us know!
I wasn’t immediately aware of this, but if you’re attempting to use HP’s QuickTest Professional (QTP) with your Flex 3 application and find that the QTP tool (during recording) causes this alert popup to display in your application:
“License not present. With the trial version only limited records are allowed.”
It is because you probably (like me) grabbed the four swc’s needed for automation and QTP from Flex Builder 3′s “sdks” folder (under frameworks/libs): automation.swc, automation_agent.swc, automation_dmv.swc and qtp.swc.
Normally this would work just fine, unless you have the Standard edition of Flex Builder. You’ll find if you upgrade to the Professional version, you’ll get access to the full licensed version of these swcs (rather than the trial versions included with the Standard edition) and the popup message will go away.
Flex Builder 3 Professional also includes a few other features that may or may not be worth the $699 price tag: http://www.adobe.com/products/flex/buy/
Recent Comments